WCF Hosting
In this part of the tutorial we are going to see the four different way of hosting the WCF service. WCF service cannot exist on its own; it has to be hosted in windows process called as host process. Single host process can host multiple servers and same service type can be hosted in multiple host process. As we discussed there are mainly four different way of hosting the WCF service.
Multiple hosting and protocols supported by WCF.Microsoft has introduced the WCF concept in order to make distributed application development and deployment simple.
Hosting Environment | Supported protocol |
---|---|
Windows console and form application | HTTP,net.tcp,net.pipe,net.msmq |
Windows service application (formerly known as NT services) | HTTP,net.tcp,net.pipe,net.msmq |
Web server IIS6 | http, wshttp |
Web server IIS7 - Windows Process Activation Service (WAS) | HTTP,net.tcp,net.pipe,net.msmq |
A summary of hosting options and supported features.
Feature | Self-Hosting | IIS Hosting | WAS Hosting |
---|---|---|---|
Executable Process/ App Domain | Yes | Yes | Yes |
Configuration | App.config | Web.config | Web.config |
Activation | Manual at startup | Message-based | Message-based |
Idle-Time Management | No | Yes | Yes |
Health Monitoring | No | Yes | Yes |
Process Recycling | No | Yes | Yes |
Management Tools | No | Yes | Yes |
IIS 5/6 Hosting
The main advantage of hosting service in IIS is that, it will automatically launch the host process when it gets the first client request. It uses the features of IIS such as process recycling, idle shutdown, process health monitoring and message based activation. The main disadvantage of using IIS is that, it will support only HTTP protocol.
Let as do some hands on, to create service and host in IIS
Step 1:Start the Visual Studio 2008 and click File->New->Web Site. Select the 'WCF Service' and Location as http. This will directly host the service in IIS and click OK.

Step 2: I have created sample HelloWorld service, which will accept name as input and return with 'Hello' and name. Interface and implementation of the Service is shown below.
IMyService.cs[ServiceContract] public interface IMyService { [OperationContract] string HelloWorld(string name); }
public class MyService : IMyService { #region IMyService Members public string HelloWorld(string name) { return "Hello " + name; } #endregion }
Step 3: Service file (.svc) contains name of the service and code behind file name. This file is used to know about the service.
MyService.svc<%@ ServiceHost Language="C#" Debug="true" Service="MyService" CodeBehind="~/App_Code/MyService.cs" %>
Step 4: Server side configurations are mentioned in the config file. Here I have mention only one end point which is configured to 'wsHttpBinding', we can also have multiple end point with differnet binding. Since we are going to hosted in IIS. We have to use only http binding. We will come to know more on endpoints and its configuration in later tutorial. Web.Config
<system.serviceModel> <services> <service behaviorConfiguration="ServiceBehavior" name="MyService"> <endpoint address="http://localhost/IISHostedService/MyService.svc" binding="wsHttpBinding" contract="IMyService"> <identity> <dns value="localhost"/> </identity> </endpoint> <endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange"/> </service> </services> <behaviors> <serviceBehaviors> <behavior name="ServiceBehavior"> <!-- To avoid disclosing metadata information, set the value below to false and remove the metadata endpoint above before deployment --> <serviceMetadata httpGetEnabled="true"/> <!-- To receive exception details in faults for debugging purposes, set the value below to true. Set to false before deployment to avoid disclosing exception information --> <serviceDebug includeExceptionDetailInFaults="false"/> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel>
You need to mention the service file name, along with the Address mention in the config file. IIS Screen shot

This screen will appear when we run the application.

Step 5: Now we successfully hosted the service in IIS. Next we have to consume this service in client application. Before creating the client application, we need to create the proxy for the service. This proxy is used by the client application, to interact with service. To create the proxy, run the Visual Studio 2008 command prompt. Using service utility we can create the proxy class and its configuration information.
svcutil http://localhost/IISHostedService/MyService.svc

After executing this command we will find two file generated in the default location.
- MyService.cs - Proxy class for the WCF service
- output.config - Configuration information about the service.
Step 6: Now we will start creating the Console application using Visual Studio 2008(Client application).

Step 7: Add the reference 'System.ServiceModel'; this is the core dll for WCF.
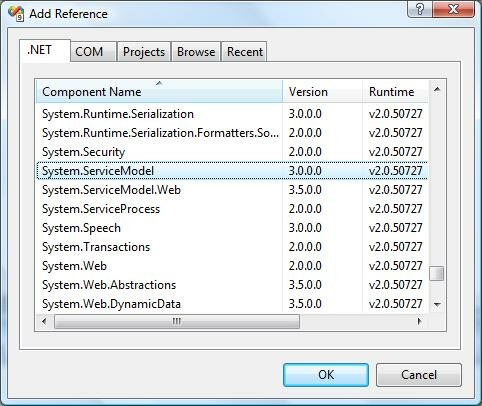
Step 8: Create the object for the proxy class and call the HelloWorld method.
static void Main(string[] args) { //Creating Proxy for the MyService MyServiceClient client = new MyServiceClient(); Console.WriteLine("Client calling the service..."); Console.WriteLine(client.HelloWorld("Ram")); Console.Read(); }
Step 9: If we run the application we will find the output as shown below.
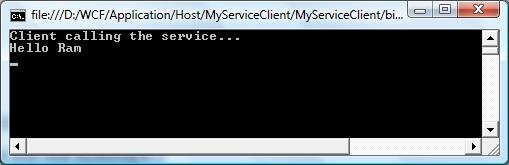
I hope you have enjoyed the Service hosted in IIS. Now let start the look on the self hosted service.
Self Hosting
In web service, we can host the service only in IIS, but WCF provides the user to host the service in any application (e.g. console application, Windows form etc.). Very interestingly developer is responsible for providing and managing the life cycle of the host process. Service can also be in-pro i.e. client and service in the same process. Now let's us create the WCF service which is hosted in Console application. We will also look in to creating proxy using 'ClientBase' abstract class.
Note: Host process must be running before the client calls the service, which typically means you have to prelaunch it.
Step 1: First let's start create the Service contract and it implementation. Create a console application and name it as MyCalculatorService. This is simple service which return addition of two numbers.

Step 2: Add the System.ServiceModel reference to the project.

Step 3: Create an ISimpleCalculator interface, Add ServiceContract and OperationContract attribute to the class and function as shown below. You will know more information about these contracts in later session. These contracts will expose method to outside world for using this service.
IMyCalculatorService.csusing System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; namespace MyCalculatorService { [ServiceContract()] public interface ISimpleCalculator { [OperationContract()] int Add(int num1, int num2); } }
Step 4: MyCalculatorService is the implementation class for IMyCalculatorService interface as shown below.
MyCalculatorService.csusing System; using System.Collections.Generic; using System.Linq; using System.Text; namespace MyCalculatorService { class SimpleCalculator : ISimpleCalculator { public int Add(int num1, int num2) { return num1 + num2; } } }
Step 5: Now we are ready with service. Let's go for implementing the hosting process. Create a new console application and name it as 'MyCalculatorServiceHost'
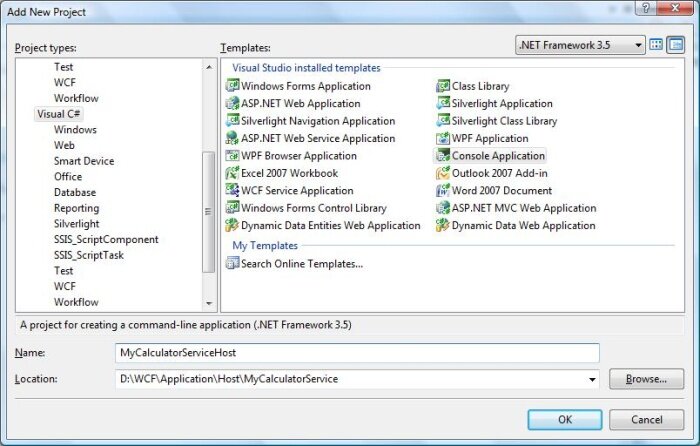
Step 6: ServiceHost is the core class use to host the WCF service. It will accept implemented contract class and base address as contractor parameter. You can register multiple base addresses separated by commas, but address should not use same transport schema.
Uri httpUrl = new Uri("http://localhost:8090/MyService/SimpleCalculator"); Uri tcpUrl = new Uri("net.tcp://localhost:8090/MyService/SimpleCalculator"); ServiceHost host = new ServiceHost(typeof(MyCalculatorService.SimpleCalculator), httpUrl, tcpUrl);
Multiple end points can be added to the Service using AddServiceEndpoint() method. Host.Open() will run the service, so that it can be used by any client.
Step 7: Below code show the implementation of the host process.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; using System.ServiceModel.Description; namespace MyCalculatorServiceHost { class Program { static void Main(string[] args) { //Create a URI to serve as the base address Uri httpUrl = new Uri("http://localhost:8090/MyService/SimpleCalculator"); //Create ServiceHost ServiceHost host = new ServiceHost(typeof(MyCalculatorService.SimpleCalculator), httpUrl); //Add a service endpoint host.AddServiceEndpoint(typeof(MyCalculatorService.ISimpleCalculator) , new WSHttpBinding(), ""); //Enable metadata exchange ServiceMetadataBehavior smb = new ServiceMetadataBehavior(); smb.HttpGetEnabled = true; host.Description.Behaviors.Add(smb); //Start the Service host.Open(); Console.WriteLine("Service is host at " + DateTime.Now.ToString()); Console.WriteLine("Host is running... Presskey to stop"); Console.ReadLine(); } } }
Step 8: Service is hosted, now we need to implement the proxy class for the client. There are different ways of creating the proxy
- Using SvcUtil.exe, we can create the proxy class and configuration file with end points.
- Adding Service reference to the client application.
- Implementing ClientBase
class
Of these three methods, Implementing ClientBase is the best practice. If you are using rest two method, we need to create proxy class every time when we make changes in Service implementation. But this is not the case for ClientBase. It will create the proxy only at runtime and so it will take care of everything.
MyCalculatorServiceProxy.csusing System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; using MyCalculatorService; namespace MyCalculatorServiceProxy { public class MyCalculatorServiceProxy : //WCF create proxy for ISimpleCalculator using ClientBase ClientBase, ISimpleCalculator { public int Add(int num1, int num2) { //Call base to do funtion return base.Channel.Add(num1, num2); } } }
Step 9: In the client side, we can create the instance for the proxy class and call the method as shown below. Add proxy assembly as reference to the project.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; namespace MyCalculatorServiceClient { class Program { static void Main(string[] args) { MyCalculatorServiceProxy.MyCalculatorServiceProxy proxy ; proxy= new MyCalculatorServiceProxy.MyCalculatorServiceProxy(); Console.WriteLine("Client is running at " + DateTime.Now.ToString()); Console.WriteLine("Sum of two numbers... 5+5 ="+proxy.Add(5,5)); Console.ReadLine(); } } }
Step 10 : End point (same as service) information should be added to the configuration file of the client application.
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <client> <endpoint address ="http://localhost:8090/MyService/SimpleCalculator" binding ="wsHttpBinding" contract ="MyCalculatorService.ISimpleCalculator"> </endpoint> </client> </system.serviceModel> </configuration>
Step 11: Before running the client application, you need to run the service. Output of the client application is shown below.
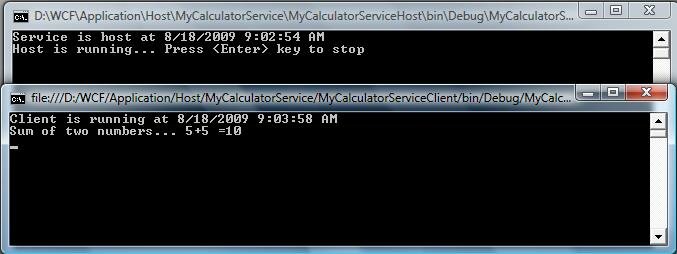
This self host shows advantage such as in-Pro hosting, programmatic access and it can be used when there need singleton service. I hope you have enjoyed the Self hosting session, now let go for hosting using Windows Activation service.
Windows Activation Service
Windows Activation service is a system service available with Windows vista and windows server 2008. It is available with IIS 7.0 and it is more powerful compared to IIS 6.0 because it supports Http, TCP and named pipes were IIS 6.0 supports only Http. It can be installed and configured separately.
Hosting WCF in Activation service takes many advantages such as process recycling, isolation, idle time management and common configuration system. WAS hosted service can be created using following steps
- Enable WCF for non-http protocols
- Create WAS hosted service
- Enable different binding to the hosted service
Enable WCF for non-http protocols
Before Start creating the service we need to configure the system to support WAS. Following are the step to configure WAS.
- Click Start -> Control Panel -> programs and Features and click 'Turn Windows Components On or Off' in left pane.
- Expand 'Microsoft .Net Framework 3.0' and enable "Windows Communication Foundation HTTP Activation" and "Windows Communication Foundation Non- HTTP Activation".
- Next we need to add Binding to the Default Web site. As an example, we will bind the default web site to the TCP protocol. Go to the Start menu -> Programs ->Accessories. Right click on the "Command Prompt" item, and select "Run as administrator" from the context menu.
- Execute the following command
- C:\Windows\system32\inetsrv> appcmd.exe set site "Default Web Site" -+bindings.[protocol='net.tcp',
bindingInformation='808:*']
Create WAS hosted service
Step 1: Next we are going to create the service, Open the Visual Studio 2008 and click New->WebSite and select WCF Service from the template and Location as HTTP as shown below.

Step 2: Create the Contract by creating interface IMathService and add ServiceContract attribute to the interface and add OperationContract attribute to the method declaration.
IMathService.csusing System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; [ServiceContract] public interface IMathService { [OperationContract] int Add(int num1, int num2); [OperationContract] int Subtract(int num1, int num2); }
Step 3: Implementation of the IMathService interface is shown below.
MathService.csusing System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; public class MathService : IMathService { public int Add(int num1, int num2) { return num1 + num2; } public int Subtract(int num1, int num2) { return num1 - num2; } }
Step 4: Service file is shown below.
MathService.svc<%@ ServiceHost Language="C#" Debug="true" Service="MathService" CodeBehind="~/App_Code/MathService.cs" %>
Step 5: In web.Config file, create end point with 'netTcpBinding' binding and service metadata will be published using Metadata Exchange point. So create the Metada Exchange end point with address as 'mex' and binding as 'mexTcpBinding'. Without publishing the service Metadata we cannot create the proxy using net.tcp address (e.g svcutil.exe net.tcp://localhost/WASHostedService/MathService.svc )
Web.Config<system.serviceModel> <services> <service name="MathService" behaviorConfiguration="ServiceBehavior"> <!-- Service Endpoints --> <endpoint binding="netTcpBinding" contract="IMathService" > </endpoint> <endpoint address="mex" binding="mexTcpBinding" contract="IMetadataExchange"/> </service> </services> <behaviors> <serviceBehaviors> <behavior name="ServiceBehavior"> <!-- To avoid disclosing metadata information, set the value below to false and remove the metadata endpoint above before deployment --> <serviceMetadata httpGetEnabled="true"/> <!-- To receive exception details in faults for debugging purposes, set the value below to true. Set to false before deployment to avoid disclosing exception information --> <serviceDebug includeExceptionDetailInFaults="false"/> </behavior> </serviceBehaviors></behaviors> </system.serviceModel>
Enable different binding to the hosted service
- Go to the Start menu -> Programs ->Accessories. Right click on the "Command Prompt" item, and select "Run as administrator" from the context menu.
- Execute the following command C:\Windows\system32\inetsrv>appcmd set app "Default Web Site/WASHostedServcie" /enabledProtocols:http,net.tcp
Output will be shown below.

Step 6: Now the service is ready to use. Next we can create the proxy class using service uttility and add the proxy class to the client application. Creat the proxy class using Visual Studio Command prompt and execute the command
svcutil.exe net.tcp://localhost/WASHostedService/MathService.svc
Proxy and configuration file are generated in the corresponding location.

Step 6: Create the client application as shown below and add the reference 'System.ServiceModel', this is the core dll for WCF.

Step 8: Add the proxy class and configuration file to the client application. Create the object for the MathServiceClient and call the method.
Program.csclass Program { static void Main(string[] args) { MathServiceClient client = new MathServiceClient(); Console.WriteLine("Sum of two number 5,6"); Console.WriteLine(client.Add(5,6)); Console.ReadLine(); } }
The output will be shown as below.

So this tutorial clearly explains about the hosting the WCF in Windows Activation Service. So next we can see how to host the service using Windows Service
Windows Service Hosting
In this tutorial we are going to see the hosting WCF service in Windows service. We will use same set of code used for hosting the WCF service in Console application to this. This is same as hosting the service in IIS without message activated. There is some advantage of hosting service in Windows service.
- The service will be hosted, when system starts
- Process life time of the service can be controlled by Service Control Manager for windows service
- All versions of Windows will support hosting WCF service.
Step 1: Now let start create the WCF service, Open the Visual Studio 2008 and click New->Project and select Class Library from the template.

Step 2: Add reference System.ServiceModel to the project. This is the core assembly used for creating the WCF service.
Step 3: Next we can create the ISimpleCalulator interface as shown below. Add the Service and Operation Contract attribute as shown below.
ISimpleCalculator.csusing System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ServiceModel; namespace WindowsServiceHostedContract { [ServiceContract] public interface ISimpleCalculator { [OperationContract] int Add(int num1, int num2); [OperationContract] int Subtract(int num1, int num2); [OperationContract] int Multiply(int num1,int num2); [OperationContract] double Divide(int num1, int num2); } }
Step 4: Implement the ISimpleCalculator interface as shown below.
SimpleCalulator.csusing System; using System.Collections.Generic; using System.Linq; using System.Text; namespace WindowsServiceHostedService { class SimpleCalculator : ISimpleCalculator { public int Add(int num1, int num2) { return num1+num2; } public int Subtract(int num1, int num2) { return num1-num2; } public int Multiply(int num1, int num2) { return num1*num2; } public double Divide(int num1, int num2) { if (num2 != 0) return num1 / num2; else return 0; } } }
Step 5: Build the Project and get the dll. Now we are ready with WCF service, now we are going to see how to host the WCF Service in Windows service. Note: In this project, I have mention that we are creating both Contract and Service(implementation) are in same project. It is always good practice if you have both in different project.
Step 6: Open Visual Studio 2008 and Click New->Project and select Windows Service.

Step 7: Add the 'WindowsServiceHostedService.dll' as reference to the project. This assembly will going to act as service.

Step 8: OnStart method of the service, we can write the hosting code for WCF. We have to make sure that we are using only one service host object. On stop method you need to close the Service Host. Following code show how to host WCF service in Windows service.
WCFHostedWindowsService.csusing System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Diagnostics; using System.Linq; using System.ServiceProcess; using System.Text; using System.ServiceModel; using System.ServiceModel.Description; namespace WCFHostedWindowsService { partial class WCFHostedWindowsService : ServiceBase { ServiceHost m_Host; public WCFHostedWindowsService() { InitializeComponent(); } protected override void OnStart(string[] args) { if (m_Host != null) { m_Host.Close(); } //Create a URI to serve as the base address Uri httpUrl = new Uri("http://localhost:8090/MyService/SimpleCalculator"); //Create ServiceHost m_Host = new ServiceHost (typeof(WindowsServiceHostedService.SimpleCalculator), httpUrl); //Add a service endpoint m_Host.AddServiceEndpoint (typeof(WindowsServiceHostedService.ISimpleCalculator), new WSHttpBinding(), ""); //Enable metadata exchange ServiceMetadataBehavior smb = new ServiceMetadataBehavior(); smb.HttpGetEnabled = true; m_Host.Description.Behaviors.Add(smb); //Start the Service m_Host.Open(); } protected override void OnStop() { if (m_Host != null) { m_Host.Close(); m_Host = null; } } static void Main() { ServiceBase[] ServicesToRun; ServicesToRun = new ServiceBase[] { new WCFHostedWindowsService() }; ServiceBase.Run(ServicesToRun); } } }
Step 9: In order to install the service we need to have the Installer class for the Windows service. So add new Installer class to the project, which is inherited from the Installer class. Please find the below code for mentioning the Service name, StartUp type etc of the service.
ServiceInstaller.csusing System; using System.Collections.Generic; using System.Text; using System.ServiceProcess; using System.Configuration.Install; using System.ComponentModel; using System.Configuration; namespace WCFHostedWindowsService { [RunInstaller(true)] public class WinServiceInstaller : Installer { private ServiceProcessInstaller process; private ServiceInstaller service; public WinServiceInstaller() { process = new ServiceProcessInstaller(); process.Account = ServiceAccount.NetworkService; service = new ServiceInstaller(); service.ServiceName = "WCFHostedWindowsService"; service.DisplayName = "WCFHostedWindowsService"; service.Description = "WCF Service Hosted"; service.StartType = ServiceStartMode.Automatic; Installers.Add(process); Installers.Add(service); } } }
Step 10: Build the project, we will get the WCFHostedWindowsService.exe. Next we need to install the service using Visual Studio Command Prompt. So open the command prompt by clicking Start->All Programs-> Microsoft Visual Studio 2008-> Visual Studio Tools-> Visual Studio Command Prompt Using installutil utility application, you can install the service as shown below.

Step 11: Now service is Hosted sucessfully and we can create the proxy class for the service and start using in the client applcaiton.
No comments:
Post a Comment